
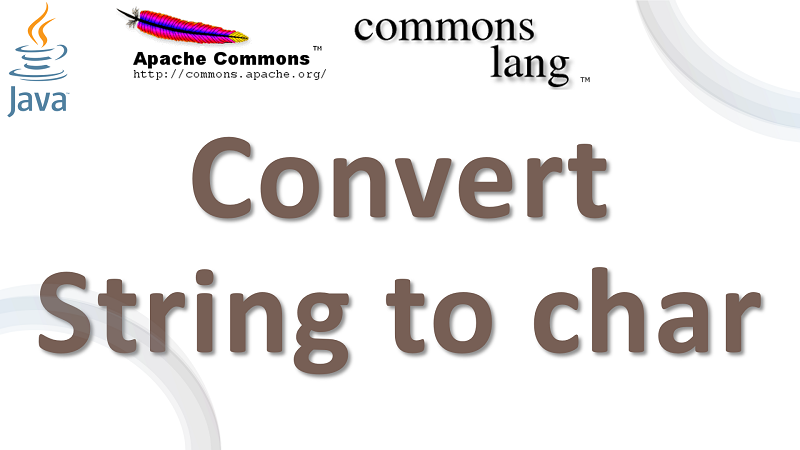

for (const char* p = x.c_str() *p ++p) *p_device = *p ) strncpy(callers_buffer, callers_buffer_size, x.c_str())), or volatile memory used for device I/O (e.g. copy x's text to a buffer specified by your function's caller (e.g.
#Convert string to char code
give "C" code access to the C++ string's text, as in printf("x is '%s'", x.c_str()).

#Convert string to char how to
So, above you've seen how to get a ( const) char*, and how to make a copy of the text independent of the original string, but what can you do with it? A random smattering of examples. Other reasons to want a char* or const char* generated from a string
#Convert string to char manual
USING malloc/free HEAP MEMORY, MANUAL DEALLOC, NO INHERENT EXCEPTION SAFETY see boost shared_array usage in Johannes Schaub's answer USING new/delete HEAP MEMORY, SMART POINTER DEALLOCATION, EXCEPTION SAFE or as a one-liner: char* y = strcpy(new char, x.c_str()) ĭelete y // make sure no break, return, throw or branching bypasses this USING new/delete HEAP MEMORY, MANUAL DEALLOC, NO INHERENT EXCEPTION SAFETY USING THE STACK TO HANDLE x OF UNKNOWN LENGTH (NON-STANDARD GCC EXTENSION) USING THE STACK TO HANDLE x OF UNKNOWN (BUT SANE) LENGTH Strncpy(y, x.c_str(), N) // copy at most N, zero-padding if shorter USING STACK WHERE UNEXPECTEDLY LONG x IS TRUNCATED (e.g. (a bit dangerous, as "known" things are sometimes wrong and often become wrong) USING STACK WHERE MAXIMUM SIZE OF x IS KNOWN TO BE COMPILE-TIME CONSTANT "N" Std::vector old_x(x.c_str(), x.c_str() + x.size() + 1) // with the NUL Std::vector old_x(x.data(), x.data() + x.size()) // without the NUL USING A VECTOR OF CHAR - AUTO, EXCEPTION SAFE, HINTS AT BINARY CONTENT, GUARANTEED CONTIGUOUS EVEN IN C++03 Given any of the above pointers: char c = p // valid for n old_x = "ab". std::string("this\0that", 9) will have a buffer holding "this\0that\0"). Even an empty string has a "first character in the buffer", because C++11 guarantees to always keep an extra NUL/0 terminator character after the explicitly assigned string content (e.g. How to get a character pointer that's valid while x remains in scope and isn't modified furtherĬ++11 simplifies things the following all give access to the same internal string buffer: const char* p_c_str = x.c_str() Ĭhar* p_writable_data = x.data() // for non-const x from C++17Ĭhar* p_x0_rw = &x // compiles iff x is not const.Īll the above pointers will hold the same value - the address of the first character in the buffer. a `char *` or `const char*` from a `string` Here, the String is converted into a character array by using the toCharArray() method.
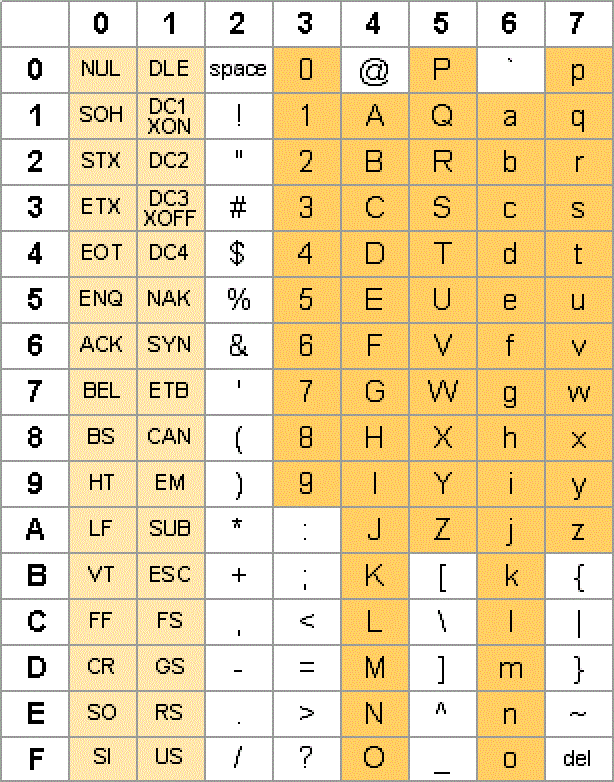
This method converts the String into a character array. The toCharArray() method is a part of String class. ("char at "+i+"th index is: "+c) Ĭhar at 4th index is: t 2. Here, the entire string is converted into character using the loop. Example 1:īy using the charAt() method, the character at a specified index is returned from the given string charAt(0) returns the first character of the String. It must be noted that this method returns only a single character and in order to get multiple characters, looping should be used. This method is used to return the single character of the specified index. The charAt() method is a part of String class. The following are the methods that will be used in this topic to convert string to char. In Java, a String can be converted into a char by using built-in methods of String class and own custom code.
